Working with DataTable in C#
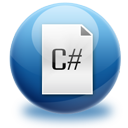
One thing I’ve had to do lately is learn some C#. I will admit that it isn’t the language I would prefer to work with during the week, but it does help me become more versatile when it comes to application development.
Working with C#, I had to take data and enter it into a data grid. Being that I still consider myself a C# newbie, I figured this was something I might as well learn to use.
In the end, creating a data table in C# isn’t that difficult. In this example, I’ll be taking data from a Microsoft Access database and entering it into a DataTable object.
First, let’s define our DataTable and its columns.
[crayon lang=”C#”]
DataTable table = new DataTable();
table.Columns.Add(“Name”, typeof(string));
table.Columns.Add(“Age”, typeof(int));
table.Columns.Add(“Weight”, typeof(int));
[/crayon]
Now, while it isn’t necessary, I like having temporary variables to hold data from a database before entering it into our table. For what we have, that would be:
[crayon lang=”C#”]
string theName;
int theAge;
int theWeight;
[/crayon]
All that is left to get is the data itself. Assuming we’re getting it from some kind of database, we can get the information in a while loop and add all of the data into the table.
[crayon lang=”C#”]
OleDbCommand cmd = new OleDbCommand(“Select FullName, PersonAge, PersonWeight FROM PersonalInfo”, con); // Our Query
OleDbDataReader reader = cmd.ExecuteReader(); // Execute Query
while (reader.Read()) // If we can read rows…
{
// Show records in text fields
theName = reader.GetString(0);
theAge = reader.GetInt32(1);
theWeight = reader.GetInt32(2);
table.Rows.Add(theName, theAge, theWeight);
}
[/crayon]
Now we have our data in the DataTable. All that is left is to output it. For this, I have a DataGridView object in my form called myDataGrid.
[crayon lang=”C#”]
myDataGrid.DataSource = table; // Put DataTable data into our DataGrid
myDataGrid.AutoResizeColumns(); // Automatically resize columns to show all data
[/crayon]
And that’s all there is to using a DataTable in C#.
Have you worked with DataTables? Submit your experiences and comments below!